Create a program that will model a music playlist by using an ArrayList
Instructions:
- Create a program that will model a music playlist by using an ArrayList that holds Song objects.
- Using jGRASP, write a Java program named LastnameFirstname14.java, using your last name and your first name, that does the following:
- Uses an ArrayList to model a music playlist.
- Uses Song objects to represent songs in the playlist, with the following instance variables:
- Title
- Artist
- Minutes
- Seconds
- Note: Your toString should print minutes and seconds together (see possible examples below in the Expected Output section)
- Create a method called createPlaylist. Create an ArrayList of at least 5 songs of your choice. Return the ArrayList.
- Create a method called editPlaylist. An ArrayList of Song objects will be passed to this method. In this method, ask the user if they would like to add or remove a song to the playlist, or if they change their mind and don’t want to add/remove any songs.
- If they choose to add a song, ask them for the song information. Create a Song object and add it to the ArrayList.
- If they choose to remove a song, print out the ArrayList in a readable format — in other words, don’t just use “System.out.println(playlist);” (see below for Example Output).
- If they change their mind and do not want to add/remove a song, don’t change anything.
- Return the ArrayList.
- Note: I recommend you use a loop here so that the user can add/remove as many songs as they like. This makes for a better, more useful program, but it is not required for this assignment.
- Create a method called savePlaylist. This playlist will have two parameters: the ArrayList and an indicator for whether the user would like to append to or overwrite a file. In the file, record the date that the playlist was saved, then write the ArrayList information to the file in a readable format — in other words, don’t just use “System.out.println(playlist);”. Confirm to the user that the file was successfully saved, and print the filename or filepath.
- In the main method:
- Call the createPlaylist method, then print the returned ArrayList. Make sure to print it out in a readable format — in other words, don’t just use “System.out.println(playlist);” (see below for Example Output).
- Ask the user if they would like to edit your playlist. If so, call the editPlaylist method and print the returned ArrayList.
- Ask the user if they would like to save the playlist. If so, call the savePlaylist method.
- Your program MUST:
- Create at least 5 Song objects, all with valid data
- Store the Song objects in an ArrayList
- Use the printPlaylist method to write out the data for all Song objects in your ArrayList to a separate text file in a neatly arranged format.
- Actively use in-line comments stating what each section of code does.
- Use try/catch if, and only if, necessary.
- Your program must conform to the Java coding standards reviewed in class during Week 3.
- Your program should not use code/concepts we have not yet covered. You must demonstrate that you have mastered the concepts covered in class.
- Remember to always begin your code with the following documentation comments:
/**
* Short description of the program.
*
* @author Last Name, First Name
* @assignment ICS 111 Assignment XX
* @date Today's Date
* @bugs Short description of bugs in the program, if any.
*/
Expected Output:
You may format your toString any way you want, as long as it presents the song info in a readable manner. Here are some suggestions:
“Say So” by Doja Cat (3:58)
“South” by Galimatias
3 minutes 35 seconds
Title: “Fly – FKJ Remix”
Artist: June Marieezy, FKJ
Time: 4:12
Feel free to choose one of these formats, or make up your own!
The following example uses the 1st format. The example also adds in the option to allow the user to continuously add/remove songs until they choose to stop, and to specify where in the playlist they’d like to add a song. These two features are optional.
----jGRASP exec: java ManuelNikki14
Hey! Check out this really cool playlist I made!
"96% of our customers have reported a 90% and above score. You might want to place an order with us."
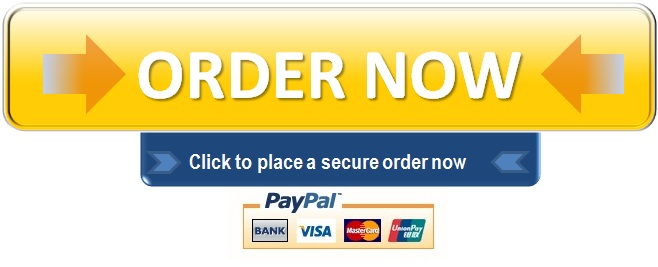