PROGRAM DESCRIPTION: In this assignment, you will be processing user submitted images in C++.
-
0
PROGRAM DESCRIPTION
VerifiedEducator
Chat
PROGRAM DESCRIPTION: In this assignment, you will be processing user submitted images in C++. Specifically, you will be labelling and counting the number of objects in a given image. Each image consists of a number of pixels, and initially all pixels will have one of two labels, 0 and1. 0 is considered a background pixel while a 1 represents a pixel that is part of an object. Your system will apply, over two iterations, the same label to pixels that are connected to form objects, and then count the number of objects for a given image.
CSCE 1030: Homework Assignment PROGRAM DESCRIPTION: In this assignment, you will be processing user submitted images in C++. Specifically, you will be labelling and counting the number of objects in a given image. Each image consists of a number of pixels, and initially all pixels will have one of two labels, 0 and 1. 0 is considered a background pixel while a 1 represents a pixel that is part of an object. Your system will apply, over two iterations, the same label to pixels that are connected to form objects, and then count the number of objects for a given image. Additionally, sample input files will be found on the CSE machines at home/jeh0289/public/csce1030/sp19/hw4 You can cd into that directory and copy the input files from there. Every line will contain the comma delimited list of 0/1 pixel labels. Example Input Image .Label 0 indicates a background pixel 11 0 1 01 0 1 11 110 00 1 Label 1 indicates a pixel that is part of an object ? ()Oly (24xmsickx visels which hares: label 1 1 0 0 1 0 0 0 0 10 1 First Pass (d) Pixel1,8) has no pixel above of left (b) Start from top left with new labelPie1,4] has no pixel above of left Create a new label 2 Create a new label 3 e) Pixell3,4) has both above and left label. Choose the smallest label Store larger label as a child of smallest (f) Completing row 7, new label 6 B) First pass complete Second Pass (c) The Final result (a) Label after First Pass (b) Converting a ‘2’ into a ‘1’ Start again from top left with new label: .checks the data structure for the label ‘1’ ‘1’ is not a child of any other labels For pixel (1,4),checks the data structure for ‘2 It notices that ‘2’ is a child of ‘1 . Then, it checks for ‘1’ (root) It replaces the label of (1, 4) with ‘1’ PROGRAM REQUIREMIENTS You must organize your program into three files: * euidHW4func.h This file will contain the include directives for the necessary libraries, any type definitions, any structure definitions, and the list of function prototypes (i.e. function euidHW4main.cpp This file will contain the local include directive for the header file as well as * the main function for the program. *euidHW4func.cpp This file will contain the local include directive for the header file as well as all function definitions (except the main) used in the program. *Be sure to replace euid with your EUID Be sure to submit all three files when submitting your assignment. As with all homework programs in this course, your program’s output will initially display the department and course number, your name, your EUID, and your email address. This output should be performed through a function you declare and define. Define a structure (i.e. struct) to represent a relationship between two integer labels. It should store two integer labels, and a pointer to another relationship structure of the same type * . * You will need to declare and define a function to process the user specified file by Prompting the user for a file name. If the file does not exist, let the user know this and reprompt until a correct file name is submitted. .Reading from the file and comectly storing the 0’s and 1’s into the dynamic 2-dimensional array You will need to declare and define a function that takes in a dynamic, 2-dimensional array and its size, and outputs the int value of every slot in a grid format. You will need to declare and define a function to perform the first pass processing of the image stored in the dynamic, 2-dimensional aray. The function should examine each pixel and process it in the following way. . . If the current pixel is a 0, the it is a background pixel and should be ignored If the current pixel is a 1, the label of the pixels above and to the left of the current pixel should be checked There will be three possible cases . Case 1: If the top and left pixels are background pixels or do not exist, create a new, non zero, positive integer, unique label and assign it to the current pixel.] ” Case 2: If one of the top or left pixels is a background pixel or does not exist, and the other has a label, the current pixel will be assigned the same label as the labeled pixel. Case 3: If both the top and left pixels have different labels, assign the current pixel the smallest label and store that there is a relationship between the two labels in a relationship structure. Be sure to connect the new structure at the end of the chain of structures, as this will be used in the second pass. See the First Pass example, and note the 2 ->1 style relations on the right side of the grid. ” ” You will need to declare and define a function to perform the second pass processing of the image stored in the dynamic, 2-dimensional array. The function should examine each pixel and process it in the following way. . If the current pixel is a 0, then it is a background pixel and should be ignored Otherwise, the pixel’s label should be checked against the chain of relationships, and if a relationship exists between its label and another label, the smaller label should replace the current one. The chain may need to be checked multiple times to ensure the smallest label is applied to a pixel. *See the Second Pass example. You will need to declare and define a function to count and output the current number of unique labels in the dynamic, 2-dimensional array Inside your main function you will need to . . Declare and dynamically allocate the correct amount of space for an 8×8, two-dimensional, dynamic array of integers representing the image to be processed. Declare a relationship structure pointer to maintain the chain of discovered relationships. Process the input file using the appropriate function and store the data in the dynamic aray. Because the dimensions of the image cannot be stored globally, be sure you store them for later function calls. Output the current state of the two-dimensional, dynamic array using the appropriate function. *Perform the first pass processing on the two-dimensional, dynamic aray using the appropriate function Output the updated state of the two-dimensional, dynamic array using the appropriate functioin. Output the current number of labels objects in the two-dimensional, dynamic array using the appropriate function. *Perform the second pass processing on the two-dimensional, dynamic array using the appropriate function and the chain of relationships. Output the final state of the two-dimensional, dynamic array using the appropriate function. Output the final number of labels objects in the two-dimensional, dynamic aray using the appropriate function. You should not to create or use any globally defined variables, though your struct should be globally defined. * . Your code should be well documented in terms of comments. For example, good comments in general consist of a header (with your name, course section, date, and brief description), comments for each variable, and commented blocks of code. This means, that in addition to the program printing your information to the terminal, it will also appear in the code in the comments as well. * Your program will be graded based largely on whether it works correctly on the CSE machines (eg.. cse01, cse02, …, cse06), so you should make sure that your program compiles and runs on a CSE machine. This programming assignment is designed to help you practice your coding on a larger project with various pieces of functionality. While the coding should be primarily your sole work, any help you I SAMPLE OUTPUT: Here is a sample output to help you write and test your code. The item in bold is the information entered by the usei $ ./a.out Computer Science and Engineering CSCE 1030 – Computer Science I Student Name EUID euid@my.unt.edu I Please enter the name of the file: imagel.txt Original image: 010 0001 0 0 10 1101 0 0 10 1101 0 010 0001 0 First pass image: 010 0001 0 0 1022 01 0 0 1022 01 0 010 0001 0 Image contains 2 objects. Second pass image: 010 0001 0 0 1022 01 0 0 1022 01 0 010 0001 0 Image contains 2 objects I $ ./a.out Computer Science and Engineering CSCE 1030 – Computer Science I Student Name EUID euid@my.unt.edu I Please enter the name of the file: imagew.txt imagew.txt does not exist. Please try again image2.txt Original image: 1 01 11011 1 11 0111 0 1 10 00 0 0 1 010 00 0 0 1 1 10 00 0 0 1 1 00 00 0 0 1 First pass image: 1 0 2 2 2 03 3 1 11 0 2 2 2 0 4 40 0 0005 0 40 00 0 0 5 6 40 00 0 0 5 6 00 00 0 0 5 Image contains 7 objects. Second pass image: 1 01 11011 1 11 0111 0 4 40 00 0 0 5 0 40 00 0 0 5 4 40 00 0 0 5 4 00 00 0 0 5 4 40 5 5 5 5 5 Image contains 3 objects. TESTING Test your program to check that it operates as desired with a variety of inputs. Then, compare the answers your code gives with the ones you get from hand calculations. Show transcribed image text CSCE 1030: Homework Assignment 4 Due: 11:59 PM on Sunday, April 28, 2019 PROGRAM DESCRIPTION: In this assignment, you will be processing user submitted images in C++. Specifically, you will be labelling and counting the number of objects in a given image. Each image consists of a number of pixels, and initially all pixels will have one of two labels, 0 and 1. 0 is considered a background pixel while a 1 represents a pixel that is part of an object. Your system will apply, over two iterations, the same label to pixels that are connected to form objects, and then count the number of objects for a given image. Additionally, sample input files will be found on the CSE machines at home/jeh0289/public/csce1030/sp19/hw4 You can cd into that directory and copy the input files from there. Every line will contain the comma delimited list of 0/1 pixel labels. Example Input Image .Label 0 indicates a background pixel 11 0 1 01 0 1 11 110 00 1 Label 1 indicates a pixel that is part of an object ? ()Oly (24xmsickx visels which hares: label 1 1 0 0 1 0 0 0 0 10 1 First Pass (d) Pixel1,8) has no pixel above of left (b) Start from top left with new labelPie1,4] has no pixel above of left Create a new label 2 Create a new label 3 e) Pixell3,4) has both above and left label. Choose the smallest label Store larger label as a child of smallest (f) Completing row 7, new label 6 B) First pass complete
Second Pass (c) The Final result (a) Label after First Pass (b) Converting a ‘2’ into a ‘1’ Start again from top left with new label: .checks the data structure for the label ‘1’ ‘1’ is not a child of any other labels For pixel (1,4),checks the data structure for ‘2 It notices that ‘2’ is a child of ‘1 . Then, it checks for ‘1’ (root) It replaces the label of (1, 4) with ‘1’ PROGRAM REQUIREMIENTS You must organize your program into three files: * euidHW4func.h This file will contain the include directives for the necessary libraries, any type definitions, any structure definitions, and the list of function prototypes (i.e. function euidHW4main.cpp This file will contain the local include directive for the header file as well as * the main function for the program. *euidHW4func.cpp This file will contain the local include directive for the header file as well as all function definitions (except the main) used in the program. *Be sure to replace euid with your EUID Be sure to submit all three files when submitting your assignment. As with all homework programs in this course, your program’s output will initially display the department and course number, your name, your EUID, and your email address. This output should be performed through a function you declare and define. Define a structure (i.e. struct) to represent a relationship between two integer labels. It should store two integer labels, and a pointer to another relationship structure of the same type * . * You will need to declare and define a function to process the user specified file by Prompting the user for a file name. If the file does not exist, let the user know this and reprompt until a correct file name is submitted. .Reading from the file and comectly storing the 0’s and 1’s into the dynamic 2-dimensional array You will need to declare and define a function that takes in a dynamic, 2-dimensional array and its size, and outputs the int value of every slot in a grid format. You will need to declare and define a function to perform the first pass processing of the image stored in the dynamic, 2-dimensional aray. The function should examine each pixel and process it in the following way. . . If the current pixel is a 0, the it is a background pixel and should be ignored
If the current pixel is a 1, the label of the pixels above and to the left of the current pixel should be checked There will be three possible cases . Case 1: If the top and left pixels are background pixels or do not exist, create a new, non zero, positive integer, unique label and assign it to the current pixel.] ” Case 2: If one of the top or left pixels is a background pixel or does not exist, and the other has a label, the current pixel will be assigned the same label as the labeled pixel. Case 3: If both the top and left pixels have different labels, assign the current pixel the smallest label and store that there is a relationship between the two labels in a relationship structure. Be sure to connect the new structure at the end of the chain of structures, as this will be used in the second pass. See the First Pass example, and note the 2 ->1 style relations on the right side of the grid. ” ” You will need to declare and define a function to perform the second pass processing of the image stored in the dynamic, 2-dimensional array. The function should examine each pixel and process it in the following way. . If the current pixel is a 0, then it is a background pixel and should be ignored Otherwise, the pixel’s label should be checked against the chain of relationships, and if a relationship exists between its label and another label, the smaller label should replace the current one. The chain may need to be checked multiple times to ensure the smallest label is applied to a pixel. *See the Second Pass example. You will need to declare and define a function to count and output the current number of unique labels in the dynamic, 2-dimensional array Inside your main function you will need to . . Declare and dynamically allocate the correct amount of space for an 8×8, two-dimensional, dynamic array of integers representing the image to be processed. Declare a relationship structure pointer to maintain the chain of discovered relationships. Process the input file using the appropriate function and store the data in the dynamic aray. Because the dimensions of the image cannot be stored globally, be sure you store them for later function calls. Output the current state of the two-dimensional, dynamic array using the appropriate function. *Perform the first pass processing on the two-dimensional, dynamic aray using the appropriate function Output the updated state of the two-dimensional, dynamic array using the appropriate functioin. Output the current number of labels objects in the two-dimensional, dynamic array using the appropriate function. *Perform the second pass processing on the two-dimensional, dynamic array using the appropriate function and the chain of relationships. Output the final state of the two-dimensional, dynamic array using the appropriate function. Output the final number of labels objects in the two-dimensional, dynamic aray using the appropriate function. You should not to create or use any globally defined variables, though your struct should be globally defined. * . Your code should be well documented in terms of comments. For example, good comments in general consist of a header (with your name, course section, date, and brief description), comments for each variable, and commented blocks of code. This means, that in addition to the program printing your information to the terminal, it will also appear in the code in the comments as well. * Your program will be graded based largely on whether it works correctly on the CSE machines (eg.. cse01, cse02, …, cse06), so you should make sure that your program compiles and runs on a CSE machine. This programming assignment is designed to help you practice your coding on a larger project with various pieces of functionality. While the coding should be primarily your sole work, any help you
I SAMPLE OUTPUT: Here is a sample output to help you write and test your code. The item in bold is the information entered by the usei $ ./a.out Computer Science and Engineering CSCE 1030 – Computer Science I Student Name EUID euid@my.unt.edu I Please enter the name of the file: imagel.txt Original image: 010 0001 0 0 10 1101 0 0 10 1101 0 010 0001 0 First pass image: 010 0001 0 0 1022 01 0 0 1022 01 0 010 0001 0 Image contains 2 objects. Second pass image: 010 0001 0 0 1022 01 0 0 1022 01 0 010 0001 0 Image contains 2 objects
I $ ./a.out Computer Science and Engineering CSCE 1030 – Computer Science I Student Name EUID euid@my.unt.edu I Please enter the name of the file: imagew.txt imagew.txt does not exist. Please try again image2.txt Original image: 1 01 11011 1 11 0111 0 1 10 00 0 0 1 010 00 0 0 1 1 10 00 0 0 1 1 00 00 0 0 1 First pass image: 1 0 2 2 2 03 3 1 11 0 2 2 2 0 4 40 0 0005 0 40 00 0 0 5 6 40 00 0 0 5 6 00 00 0 0 5 Image contains 7 objects. Second pass image: 1 01 11011 1 11 0111 0 4 40 00 0 0 5 0 40 00 0 0 5 4 40 00 0 0 5 4 00 00 0 0 5 4 40 5 5 5 5 5 Image contains 3 objects. TESTING Test your program to check that it operates as desired with a variety of inputs. Then, compare the answers your code gives with the ones you get from hand calculations.
7 days ago
Purchase the answer to view it
-
PixelPass3.zip
- Home
- Homework Answers
- Blog
- Archive
- Tags
- Reviews
- Contact
-
"96% of our customers have reported a 90% and above score. You might want to place an order with us."
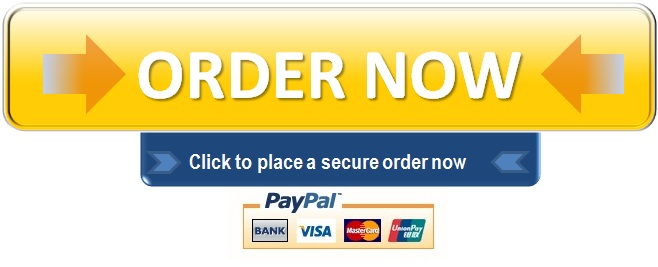