Exercise : Write a program in C or C++ or Java to display the following information.
1. Time information.
In this section you will print out how long the system has been up and how busy it has been. Once you have some baseline numbers printed, you will run a short program that places a load on the system. You will then take a second set of numbers and calculate the load that your program placed on the system.
a. Duration of uptime # get these information from /proc/uptime
b. Duration of idletime
Calculating load average.
Write a function that does some work (to put some load on the system) and make a note of the uptime and idletime before and after the call of the function. The following can be used as sample code for the work function. This program simple runs a math calculation a large number of times, just trying to keep the CPU busy. You can include it as a function in your overall program. Note that because you are using a math function (pow) you will need to explicitly include the math library when you compile your program, i.e., “gcc –o test test.c –lm”. (the –lm option for program compiling in C or C++.)
void work()
{
double y;
double x = 3.0;
double e = 2.0;
int i,j;
for (i = 0; i < 5; i++)
{
for (j = 0; j < 400000; j++)
{
y = pow(x, e);
}
printf(“Loop %d of work cyclen”, i );
// pause for one second between loops so that the work cycle takes a little time.
sleep (1); // in C or C++ you will need to include the unistd.h library for this function
}
}
The skeleton of the program
(1) read file “/proc/uptime” to obtain beginTotaltime and beginIdletime
(2) call work( ) to put some work into the system
(7) read file “/proc/uptime” to obtain endTotaltime and endIdletime
(8) Calculate the percentage of the time that CPU was busy during this program:
programTotalTime = endTotalTime – beginTotalTime;
programIdleTime = endIdleTime – beginIdleTime;
programWorkTime = programTotalTime – programIdleTime;
percentage = (programWorkTime / programTotalTime)* 100;
Items to turn in to Week 7 dropbox:
1. Source code with filename (linuxtime.c or linuxtime.cpp or linuxtime.java)
2. Sample output from your program
NOTE: (IMPORTANT !!!)
1. All the source file should be with enough documentation either embedded in the code or in a separate file. Insufficient documentation leads to minus points.
2. Be sure to write your name, date, assignment name and a brief description of the project on the top of the file in comments.(All of this counts towards the documentation)
3. Use the same file name as mentioned above (linuxtime.c or linuxtime.cpp or linuxtime.java) and submit it to Week 7 dropbox.
Percentage Distribution
Total : 100%
• Execution of the program with required output : 70%
• Enough Documentation : 20%
• Submitting the sample output : 5%
• Using the same file name as mentioned above : 5%
Save it as uptime.c
Compilation, as stated before: gcc -lm -o uptime uptime.c
Running: ./uptime
Here are my results:
Loop 0 of work cycle
Loop 1 of work cycle
Loop 2 of work cycle
Loop 3 of work cycle
Loop 4 of work cycle
Total time: 8.78 seconds
CPU idle time : 5.09 seconds
CPU busy time : 3.69 seconds
CPU % of time busy/total : 42%
"96% of our customers have reported a 90% and above score. You might want to place an order with us."
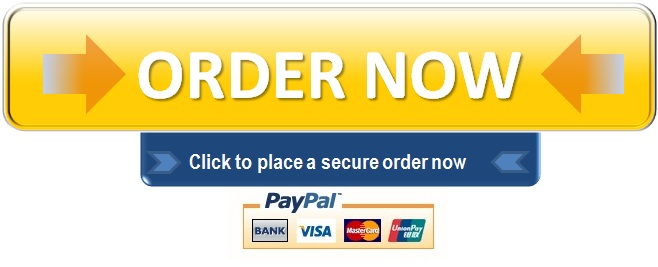