// Define a list of possible usernames
var usernames = [“admin”, “root”, “support”, “user”];
// Define a target username
var targetUsername = “admin”;
// Loop through the list of usernames and try to guess the target username
for (var i = 0; i < usernames.length; i++) {
if (usernames[i] === targetUsername) {
console.log(“Username found: ” + targetUsername);
break;
}
}
// Define a list of possible passwords
var passwords = [“password123”, “qwerty”, “letmein”, “monkey”, “12345678”];
// Define the password to be cracked
var targetPassword = “qwerty”;
// Loop through the list of passwords and try to guess the target password
for (var i = 0; i < passwords.length; i++) {
if (passwords[i] === targetPassword) {
console.log(“Password found: ” + targetPassword);
break;
}
}
for (var i = 1; i <= 65535; i++) {
var socket = new WebSocket(“ws://” + i);
socket.onopen = function() {
console.log(“Port ” + i + ” is open”);
}
}
"96% of our customers have reported a 90% and above score. You might want to place an order with us."
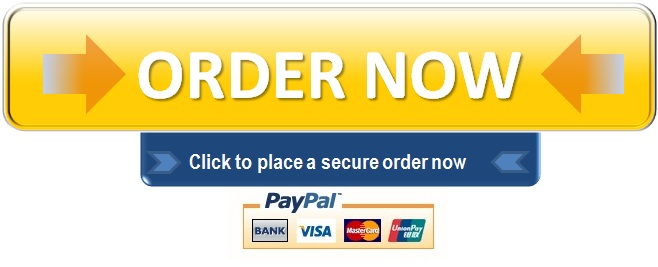